14.5. The Xcode Debugger If Script Editor is the hamburger of AppleScript, Xcode is the two-pound steak. It's fatter, heavier, and more tender in the middle than the humble Script Editor. In all seriousness, though, Xcode is a power user's dream compared to Script Editor. Although most people use Xcode for writing full-fledged programs (Section 15.2.1), there's nothing to keep you from using Xcode to write and debug your scripts. In fact, Xcode has a number of features that simply aren't available in Script Editorfeatures that can save you time, trouble, and tons of repetitive debugging. First, however, you have to install Xcode.  | If you're a feature fiend, be sure to check out third-party AppleScript editors like Smile and Script Debugger. Those programs include even more debugging features than Xcode, as detailed on Sidebar 2.6. |
| 14.5.1. Obtaining and Installing the Developer Tools Xcode is part of a powerful package for programmers (say that five times fast). Depending on whom you ask, this package is known as either the Developer Tools or the Xcode Tools. Either way, you have to install these tools to use Xcode. Gem in the Rough The error Command | When you want to handle an error in your script, try and error statements are your best friends. Sometimes, however, you want to create an error messagewhen your script encounters an unknown file, for example. In these situations, you'll be glad to have the error command, which lets you create (or, in nerd-ese, raise) your own error messages. The error command (not to be confused with error statements, which are meant to handle errors) works very similarly to the log command. Unlike log, however, the error command displays an error dialog box and interrupts your script. Therefore, the error command's use is mainly limited to critical sections of code, like the following: tell application "Finder" if not (exists the folder ¬ "Applications" ¬ of the startup disk) then error ¬ "Your Applications folder is gone!" end if end tell When you run that script, AppleScript checks whether you have an Applications folder. If you don't, the script presents the dialog box shown here, and stops your script in its tracks. 
Of course, you can use error statements (Section 14.4.2) in conjunction with the error command. That way, you can trap problems that would otherwise interrupt your script, and instead log a message, or have your script do nothing at all. The power of these two debugging toolserror commands for creating error messages and error statements for suppressing themopens up a world of opportunity for any scripter with buggy code. |
If you bought the retail, boxed version of Mac OS X, the Xcode Tools came on a special CD of their very own. If so, you have it easy: simply insert the CD and follow the instructions in the included help document. On the other hand, if Mac OS X came with your Mac, you may not have received the Xcode Tools on a disc. In that case, you have it harder: Visit http://connect.apple.com/, and click Join ADC. The ADC (or, as geeks would have it, Apple Developer Connection) is a club for Mac programmers. Once you've joined (the simplest membership"Online"is free), you can download various programs and sample code. Click Agree to accept Apple's terms of membership. Basically, the agreement boils down to two parts: you are who you say you are, and you won't go telling people trade secrets. Provide all the information Apple requests, and click Continue when you're done. You should receive a welcome email after you've set up your account. Once your account is active, return to http://connect.apple.com/, and log in. If you're using a Web browser like Safari, you can even have Mac OS X remember your user name and password for future use. Click Download Software. In the submenu that appears, click Mac OS X. You see a list of updates to the Xcode Tools. Download the most recent revision of the tools. Be patient, though: the download can be more than 350 MB, so it may take several hours to download, even over a high-speed Internet connection. Install the Xcode Tools from the disk image you just downloaded. A disk image is a virtual recreation of a disk. With the tools you just downloaded, for example, the disk image contains what you'd see if you had an actual CD with the Xcode Tools on it. Once you install the tools successfully (and restart your Mac), you're ready to go. Launch Xcode from your Developer Applications folder. 14.5.2. Creating a New Project Unlike Script Editor, Xcode creates new files as projects. A project is, in essence, a cluster of related files meant to be edited and run as a group. To use Xcode's powerful AppleScript tools, therefore, you have to create a new project. Luckily, that's a pretty simple procedure: Choose File New Project, or press Shift- -N. You'll see the dialog box shown at the top of Figure 14-7. Choose AppleScript Droplet and click Next. An AppleScript Droplet automatically comes with run and open handlers, which you can fill in with any code you please.  | If you don't need either of these handlers in your project, you can choose AppleScript Application instead. Still, for the purposes of this example, you will use the idle handler, so be sure to choose AppleScript Droplet. |
|
Give your project a name, such as Debugaroo, and click Choose to specify where you want to save the project. Click Finish to create the project in the location you specified (Figure 14-7, bottom). Now you have a full-fledged Xcode project, just awaiting some of your code.  | Technically, what you just created was an AppleScript Studio project. That means, if you wanted to, you could create a full-fledged graphical interface for your script (Section 15.2.2). Still, if all you want to do is debug a script, all these graphical capabilities are downright distracting. For the rest of this chapter, therefore, you'll stick with the nongraphical side of AppleScript. (But if you do want to learn more about creating graphical interfaces for your scripts, just skip ahead to Chapter 15.) |
| 14.5.3. Changing the Script Of the myriad folders in the project before you, only the Scripts folder contains an AppleScript file. To add code to your project, you have to open this filenamed, creatively enough, Application.applescript, and make your modifications there (Figure 14-8). Say you wanted to make a program that saved all your TextEdit documents every 10 minutes. Since Xcode already created an idle handler for you, all you have to do is fill it in: on idle tell application "TextEdit" --Get a list of unsaved documents: set unsavedDocs to every document whose modified is true --Count them up: set numberOfUnsavedDocs to (count unsavedDocs) --Make sure there *are* unsaved documents if numberOfUnsavedDocs is not 0 then --Save the documents one at a time: repeat with currentDoc in unsavedDocs save currentDoc end repeat end if end tell --Come back and check if there are any unsaved documents in ten minutes return 600 end idle  | Although your Xcode project also includes an open handler, there's no need to fill it in with any code. For this script, only the idle handler is of any use (although some scripts do use open handlers for operating on a group of files). |
| 14.5.4. Stepping Through the Code Now, all the commands from that script are pretty straightforward. The only problem is, you have no way of knowing when the script is running each command. This, unfortunately, is a problem with all AppleScripts: there's no way of stepping through a script command by command. At least, that would be your feeling if you were trained on Script Editor. Don't despair, thoughXcode can take over the job where Script Editor left off: by letting you step through your code. Before you can do that, though, you have to set breakpoints in your script. Basically, breakpoints tell Xcode, "When you run my script and hit one of these lines, pause the script." To set breakpoints, simply click in the left gutter of your script window (Figure 14-9). 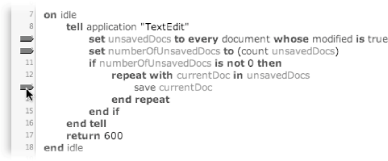 | Figure 14-9. Here, you're setting a breakpoint on line 13. When you run your script, Xcode will pause and bare its AppleScript guts when it runs the command on line 13. |
|
Once you've set breakpoints on the lines that interest you, choose Build Build and Debug ( -Y) to get your script cooking. Xcode prompts you to save your project first, so just go ahead and click Save All. As soon as Xcode encounters a line for which you've set a breakpoint, it stops. As an added bonus, Xcode displays all sorts of technical information: the values of your variables, the handler that's currently running, and other statistics that only a geek could love (Figure 14-10).  | Amazingly, you can actually change the values of your variables while your script is running! Simply double-click in the Value column and enter the new value you want to use. You might find this trick useful if, for example, you accidentally misspell a response in a dialog box. Rather than restarting your entire script, you could simply open the Debugger, double-click the variable that stores your response, correct the typo, and let your script continue running. On the other hand, don't get too carried away with the ability to change variables while your script is running. Since the Debugger won't stop you from replacing a number with a string, for example, there's nothing to keep you from seriously messing up your variables. In the worst case, tweaking your variables could lead your entire script to crash. |
| 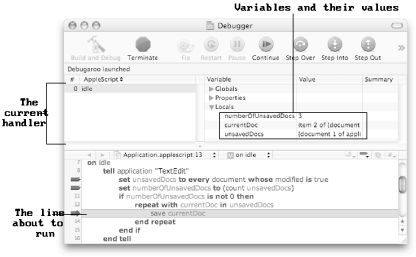 | Figure 14-10. The magic that is Xcode's Debugger. To jump to the next breakpoint, click Continue. Or, if you've had enough of the intoxicating Debugger power, click Terminate to stop your script. |
|
This script is but one example of debugging in action. For real fun, try taking a big, complicated script and copying it into an Xcode project. Set a few breakpoints, get the Debugger going, andVoila!your script pauses for inspection at each breakpoint.  | If you want to know what a script is doing at the momenteven if it isn't at a breakpointclick the Pause button in the Debugger. Xcode locates the command that's currently running, and highlights it for your inspecting pleasure. |
| Finally, if none of the tricks in this chapter can fix a certain problem, be sure to check out Appendix C. There, you'll find a list of books, Web sites, and email lists for people with scripting problems. |